Warning: You are viewing an older version of this documentation. Most recent is here: 40.0.1
REST API¶
The REST API provides a way to interact with Stamus Central Server (SCS) and registered Probes programmatically through HTTP requests using JSON format.
Generate an Access Token¶
To access the REST-API, a user first need to generate a unique token associated to its account. The API accesses are made on the behalf of a given user.
To do that, login to SCS and go under your account settings from the header’s menu.
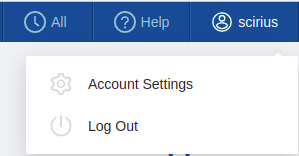
From the left side panel, under User Settings, select Edit Token.
If you already generated a token for this account, it will be presented in the Token field, otherwise this field will be empty.
In both cases, to generate a new token, simply click the Regenerate button at the bottom of the page and you should see the Token field updated with a hash value such as 3064d9deadbeef36436daba5531e105123ec0fee.
Accessing the REST-API¶
To access the REST-API, one can choose to access it programmatically using the command cURL for example, or browse the REST-API endpoints through the Web Interface.
An access to the REST-API using command line will look like the following:
curl -X GET -k https://<SCS_ADDRESS>/rest/<ENDPOINT>/<OPTIONAL_PARAMETERS> -H 'Authorization: Token <TOKEN>' -H 'Content-Type: application/json'
To access the REST-API:
The HTTP header
Content-Type: application/json
must be providedThe Access Token must be sent using the HTTP header
Authorization: Token <TOKEN>
The body of the request must contain JSON parameters encoded in UTF8 (if the request supports parameters)
To access the Web Interface of the REST-API, simply open your browser to https://<SCS_ADDRESS>/rest/
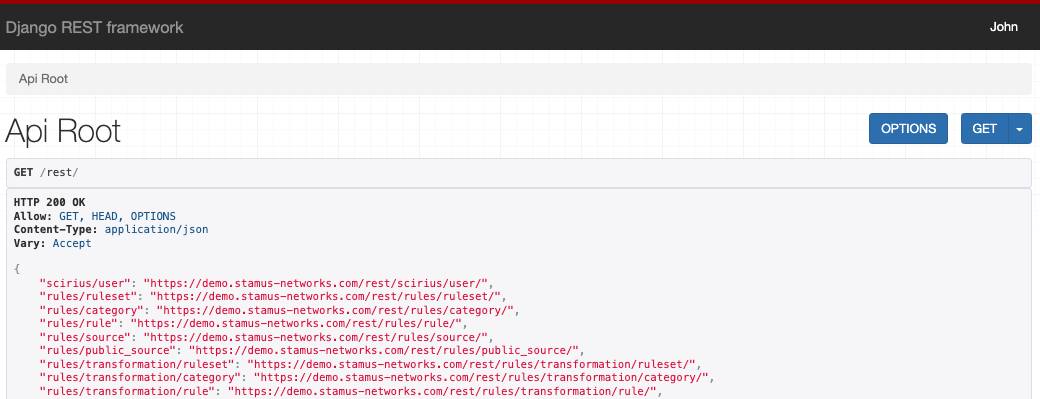
API usage¶
HTTP Methods¶
The REST-API allows accessing different kind of objects such as user accounts, rulesets, sources, threats and so on. Each object has its own endpoint and the following table summarize the different kind of accesses that can be made.
In this table, pk represent the Primary Key (unique identifier) of a given object.
URL |
HTTP Method |
JSON Parameters |
Description |
---|---|---|---|
|
GET |
List all objects of type <object> |
|
|
POST |
Dictionary of key/value of field |
Create an object of type <object> |
|
OPTIONS |
Description of the fields of the object |
|
|
GET |
Returns all the fields of the object |
|
|
OPTIONS |
Description of the fields of the object |
|
|
PUT |
Dictionary of key/value of field |
Update the fields specified in parameters |
|
PATCH |
Dictionary of key/value of field |
Like PUT, but skips checking for the
presence of |
|
DELETE |
Deletes the object |
|
|
GET/POST |
Dictionary describing the action (on POST request) |
Performs an <action> related to <object> |
|
GET/POST |
Dictionary describing the action (on POST request) |
Performs an <action> on the object |
Hint
Most queries accept an optional dictionary with a comment
key to be stored in the actions history.
List Parameters¶
When using the GET method to retrieve a list of objects, the following parameters may come handy to navigate the data retrieved. Those parameters are also available through the Web Interface from the Filters dropdown.
Type |
Parameter |
Description |
---|---|---|
Pagination |
|
Specify the page number to retrieve. Each call returns at most 30 results |
Ordering |
|
Results can be sorted ascendingly by specifying the field name, or descendingly by preceeding the field name with |
Searching |
|
List of comma-separated or space-separated keywords. Results returned contain all keywords specified, matched case insensitively. |
Filtering |
|
Filtering allows to filter by field on exact matches (for character strings, the whole text has to match, case sensitive). Multiple filters can be combined. |
Accessing Host ID¶
Stamus’ unique capability Host ID is also accessible through the REST-API and when used, one gets the very same information as shown in Stamus Central Server.
The following endpoint, /rest/appliances/host_id/<IP_ADDRESS>
, will retrieve all Host ID information available for the specified IP address.
Examples¶
cURL¶
curl -X GET -k https://<SCS_ADDRESS>/rest/appliances/host_id/<IP_ADDRESS> -H 'Authorization: Token <TOKEN>' -H 'Content-Type: application/json'
For example, if your SCS IP address is 10.11.0.12
and you want to get more information on 10.0.0.101
, the query will look like the following:
curl -X GET -k https://10.11.0.12/rest/appliances/host_id/10.0.0.101 -H 'Authorization: Token 3064d9deadbeef36436daba5531e105123ec0fee' -H 'Content-Type: application/json'
Python¶
import requests
headers = {'Authorization': 'Token <TOKEN>', 'Content-Type': 'application/json'}
res = requests.get('https://<SCS_ADDRESS>/rest/appliances/host_id/<HOST_IP>', params={}, verify=False, headers=headers)
print(res.text)
Direct Link¶
You could also use a direct web url to open up HostID web GUI page for an IP:
https://<SCS_ADDRESS>/rules/hunt?session=temporary&page=HOSTS_LIST&ip=<IP_ADDRESS>
For example, if your SCS IP address is 10.11.0.12
and you want to get more information on 10.0.0.101
, the link should look like the following:
https://10.11.0.12/rules/hunt?session=temporary&page=HOSTS_LIST&ip=10.0.0.101
Objects relations¶
SCS and probes¶
A few addresses describe probes, with slight differences that can be useful depending on the use case:
/rest/appliances/stamus_probe/
contains all Stamus probes with parameters writable./rest/appliances/stamus_probe_template/
contains all Stamus probe templates with parameters writable./rest/appliances/suricata_probe/
contains all Suricata probes with parameters writable./rest/appliances/appliances/
lists all Stamus probes and Suricata probes read-only (it mainly contains fields common to Stamus and Suricata probes, plus atype
field which can be eitherStamus
orSuricata
)./rest/appliances/stamus_device/
contains all Stamus probes and SCS read-only (SCS always has apk
of 1).stamus_probe
objects contain astamusdevice_id
field that corresponds to the primary key of thestamus_device
object.
Primary keys of stamus_probe
, suricata_probe
and appliance
objects are shared.
That is, the pk
of an appliance
object can be combined to the type
field to find the
address of the corresponding full object.
Network interfaces¶
Network interfaces objects are tied to Stamus probes and probes templates. Interfaces are added by
setting the interfaces
field of stamus_probe
and stamus_probe_template
. This
field can be set with a list of interfaces defined by a dictionary {"name": "eth0", "active": true/false}
.
When reading the interfaces
field, the interfaces dictionaries will contain the pk
of the
interface object available at /rest/appliances/network_interface/
. Interfaces can be deleted
by a DELETE
to the network_interface
object, or by changing the interfaces
fields of the
probe or template.
Protocols¶
Protocols work the same way as interfaces using the protocols
fields of a probe or a template.
Templates¶
Stamus probes (and templates) can inherit a template by setting the parent_tmpl
field of a
stamus_probe
to the value of the pk
of the stamus_probe_template
. Subsequent
modifications to the template are then applied automatically to its children objects.
To override a field of an object that inherits from a template, it is necessary to list it in
the overriden_fields
field of this object.
Inheritance works the same way for interfaces.
However, protocols use the detection
key, instaed of active
. Thus, if we have a parent template with a child template and
enable the child template on a SN Probe, any modifications to the protocols
of the parent template will not be reflected on the child
child template.
User actions¶
/rest/rules/history/
contains user actions that were performed on the SCS.
User actions entries contain a description_raw
field that is a template of the description with
pointers to objects (the rendered description is available in the description
field).
The identifiers enclosed in curly brackets in description_raw
are defined in the ua_objects
field.
Some ua_objects
can be linked to an existing object (rule, probe …) using its type
and pk
(or sid
for rules). In case the object was deleted, the pk
is not present. Other objects
(transformation, …) only contain the value
field.